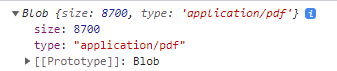
[Java/Javascirpt] Uint8Array to PDF file 변환하기 (front to Back File io)
리트리버J
·2022. 5. 9. 16:56
728x90
PDF파일을 HTML Canvas를 이용해 서명이나 텍스트, 사진등을 추가하여
변경된 PDF 파일을 업로드를 해야만 했다.
1. PDF -> BLOB
먼저, 어떤 pdf 파일을 fetch API를 통해 불러온 후,
blob() 함수를 이용해 형변환 해준다.
1
2
3
|
const res = await fetch("test.pdf");
const pdfBlob = await res.blob();
console.log(pdfBlob);
|
cs |
Blob 한 결과를 화면에 뿌려주어 CANVAS를 통해 원하는 PDF로 만들고
1
2
3
4
5
6
7
|
export async function readAsPDF(file) {
const pdfjsLib = await getAsset('pdfjsLib');
// Safari possibly get webkitblobresource error 1 when using origin file blob
const blob = new Blob([file]);
const url = window.URL.createObjectURL(blob);
return pdfjsLib.getDocument(url).promise;
}
|
cs |
2. BLOB -> ArrayBuffer(Uint8Array)
저장 할 때 ArrayBuffer(Uint8Array) 로 만들어주었다.
1
2
3
4
5
6
|
try {
pdfDoc = await PDFLib.PDFDocument.load(await readAsArrayBuffer(pdfFile));
} catch (e) {
console.log('Failed to load PDF.');
throw e;
}
|
cs |
3. ArrayBuffer(Uint8Array) -> BLOB
ArrayBuffer (Uint8Array)를 Java Spring에서 처리 하려니 라이브러리도 잘 없고
여간 복잡해서
Svelte Fornt에서 Blob형태로 담아서 보내주었다.
CORS 문제가 있으니 fetch 설정의 no-cors를 해주어야 한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
try {
// 1. Uint8Arrays 형태로 들어감
console.log(pdfBytes);
/* 2. 업로드
2-1. FormData 생성*/
const dataArray = new FormData();
const arr = new Uint8Array(pdfBytes);
const blob = new Blob([arr], { type: 'application/pdf' });
console.log(blob);
// 2-2. FormData에 Data 추가
dataArray.append("pdfBytes", blob);
dataArray.append("name", name+" sample");
// 2-3. fetch 실행
fetch("http://localhost:8082/pdfDownload", {
mode : 'no-cors',
method: "POST",
headers: [["Content-Type", "application/json"]],
body: dataArray
})
.then((response) => {
console.log(response);
})
.catch((error) => {
console.log(error);
});
} catch (e) {
console.log('Failed to save PDF.');
throw e;
}
|
cs |
4. BLOB -> PDF
fornt fetch에서 POST로 보냈으니 @PostMapping
Blob형태는 MultipartFile로 받을 수 있었다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
@RestController
public class DownloadController {
@PostMapping("/pdfDownload")
public String pdfDownload(HttpServletResponse response, @RequestParam Map<String, Object> map, @RequestParam MultipartFile pdfBytes){
// 1. reponse 정보
System.out.println(response);
// 2. blob 데이터 (MultipartFile)
System.out.println(pdfBytes.getClass());
// 3. File로 변환 후 저장
try {
// 3-1. 이름 설정
FileOutputStream out = new FileOutputStream(map.get("name") + ".pdf");
// 3-2. 파일 저장
out.write(pdfBytes.getBytes());
// 3-3. 버퍼 출력
out.close();
}catch(Exception e) {
e.printStackTrace();
}
return null;
}
}
|
cs |
728x90